LINQ stands for Language Integrated Query is a Microsoft .NET Framework component that adds data querying capabilities to .NET languages. It was introduced in Visual Studio 2008 as a major part of .NET Framework 3.5 in 2007 and it was designed by Anders Hejlsberg. LINQ extends the language by the addition of query expressions, which are similar to SQL statements and can be used to conveniently extract and process data from collections (such as arrays, and enumerable classes), XML documents, relational databases, and third-party data sources.
What is LINQ?
LINQ stands for Language Integrated Query and it allows you to query data from different data sources such as arrays, enumerable classes, XML documents, relational databases, and third-party data sources by using a uniform API and syntax.
LINQ Architecture
LINQ is not a specific architecture by itself, but rather a set of technologies and features integrated into the .NET Framework that provides a consistent query experience across various data sources. LINQ enables developers to query different types of data using a unified syntax, whether the data is in-memory collections, databases, XML, or other formats.
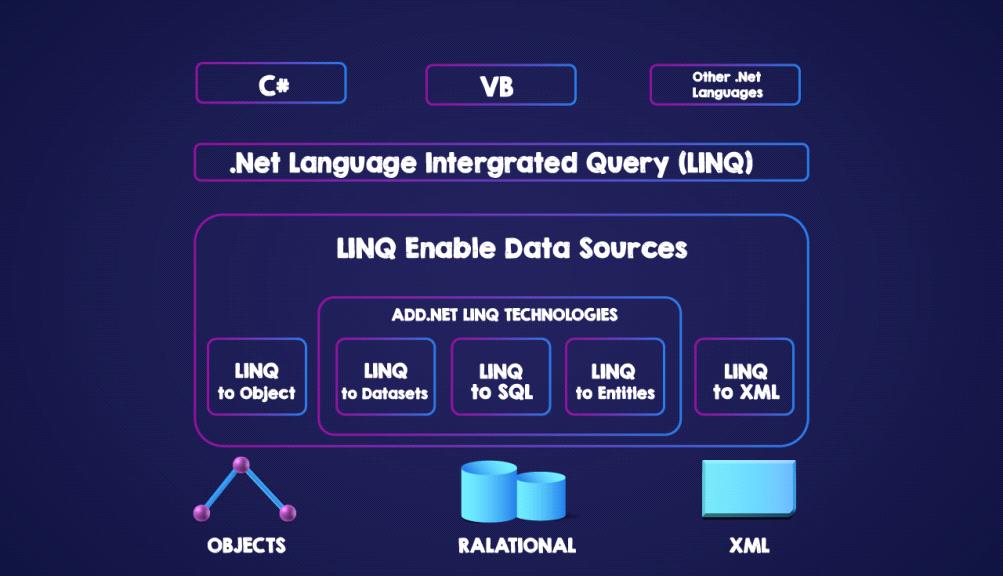
The primary components and features of LINQ include:
1. LINQ Providers: LINQ introduces the concept of providers, which are responsible for interpreting and executing LINQ queries against specific data sources. Different LINQ providers are available for different types of data sources, such as LINQ to Objects, LINQ to SQL, LINQ to XML, LINQ to Entities, and more.
2. Standard Query Operators: LINQ provides a set of standard query operators, which are methods defined in the System.Linq namespace. These operators, such as Where, Select, OrderBy, GroupBy, etc., allow developers to perform common query operations on data sources.
3. Query Expressions: Developers can use query expressions to write LINQ queries in a declarative syntax that resembles SQL. These expressions are translated into method syntax by the compiler, allowing developers to express queries more naturally.
var query = from item in collection
where item.Property > 10
select item;
4. Lambda Expressions: Lambda expressions are used extensively in LINQ to define anonymous functions. These expressions often appear in the form of predicate functions, projections, or other delegate-based operations.
var result = collection.Where(item => item.Property > 10).Select(item => item.Name);
5. LINQ to Objects: LINQ to Objects enables querying in-memory collections (such as arrays, lists, and dictionaries) using the standard query operators.
6. LINQ to SQL and LINQ to Entities: LINQ to SQL and LINQ to Entities are LINQ providers designed for querying relational databases. They allow developers to write LINQ queries against database tables and execute them efficiently.
7. LINQ to XML: LINQ to XML provides a way to query and manipulate XML data using LINQ. It includes standard query operators for working with XML elements and attributes.
8. Extension Methods: LINQ relies on extension methods to implement the standard query operators. These methods extend the functionality of existing types to support LINQ queries.
Overall, LINQ simplifies and unifies the process of querying different types of data sources in a type-safe manner. Developers can use LINQ to express complex queries with a consistent syntax, making code more readable and maintainable. The specific architecture and implementation details can vary based on the LINQ provider being used (e.g., LINQ to SQL, LINQ to Objects, etc.).
What are the Advantages of using LINQ?
Using LINQ (Language-Integrated Query) in your C# code provides several advantages, making it a powerful tool for data manipulation and querying. Here are some of the main advantages of using LINQ:
- Unified Query Syntax: LINQ provides a unified syntax for querying different types of data sources, such as in-memory collections, databases, XML, and more. This consistency simplifies the code and reduces the learning curve for developers.
- Type Safety: LINQ queries are strongly typed, which means that the compiler checks for type compatibility at compile time. This helps catch errors early in the development process and promotes safer code.
- Readability and Maintainability: LINQ queries use a declarative syntax that closely resembles SQL, making code more readable and expressive. This improves code maintainability and reduces the likelihood of introducing errors.
- Code Reusability: LINQ promotes the use of modular queries through the creation of reusable components. Developers can encapsulate complex queries into methods or functions, enhancing code reusability.
- IntelliSense Support: LINQ queries benefit from IntelliSense, providing developers with auto-completion suggestions and information about available methods and properties during development. This feature helps in writing more accurate and efficient queries.
- Easier Debugging: LINQ queries are debuggable, and developers can set breakpoints, step through code, and inspect variables just like any other C# code. This simplifies the debugging process for complex queries.
- Optimization and Deferred Execution: LINQ queries are often optimized by the underlying LINQ providers, resulting in efficient execution plans. Additionally, LINQ queries use deferred execution, meaning they are executed only when the result is needed, improving performance.
- Integration with Existing Code: LINQ can be seamlessly integrated with existing code and objects. This allows developers to leverage LINQ in projects without requiring significant changes to the overall project architecture.
- LINQ to SQL and LINQ to Entities: LINQ provides LINQ to SQL and LINQ to Entities for easy integration with relational databases. These providers generate efficient SQL queries based on LINQ expressions, providing a convenient way to interact with databases.
- Parallel LINQ (PLINQ): PLINQ allows for parallel execution of queries, taking advantage of multi-core processors and improving performance for data-intensive operations.
- LINQ to XML: LINQ to XML simplifies working with XML data, providing a concise and expressive way to query, create, and manipulate XML documents.
By combining these advantages, LINQ facilitates more efficient and expressive code for querying and manipulating data, making development tasks more straightforward and maintaining a higher level of code quality.
What are the disadvantages of using LINQ?
While LINQ (Language-Integrated Query) offers many advantages, it's important to consider potential disadvantages as well. The drawbacks of using LINQ are generally related to specific scenarios and requirements. Here are some potential disadvantages of using LINQ:
- Learning Curve: Learning LINQ might take some time, especially for developers who are new to it. The syntax and concepts, like query expressions and lambda functions, can be a bit challenging initially.
- Performance Concerns: There's a slight performance cost associated with LINQ due to its abstraction layer. For critical performance scenarios, developers might need to carefully consider alternatives or optimize LINQ queries.
- Debugging Complexity: Debugging complex LINQ queries can be a bit like untangling a knot. It's not always as straightforward as debugging traditional loops, and understanding what's happening in the middle of a LINQ chain might take some extra effort.
- Compatibility Challenges: LINQ might not always play well with certain libraries or code written in a non-LINQ style. There can be integration challenges, especially in projects with existing codebases that weren't originally designed with LINQ in mind.
- Deferred Execution Surprises: The idea of deferred execution, while powerful, can be a bit tricky. Developers need to be mindful of when a LINQ query is actually executed, especially when there are changes to the underlying data source.
- Complexity for Simple Queries: For simple queries, using LINQ may introduce unnecessary complexity. Traditional looping constructs might be more straightforward in such cases.
- SQL Optimization Challenges: When using LINQ to interact with databases, developers might have limited control over the generated SQL queries. This lack of control can be a concern when aiming for optimal database performance.
- Compatibility with Non-LINQ Code: LINQ code may not always seamlessly integrate with existing non-LINQ code or libraries. Some third-party libraries may not support LINQ, or there may be compatibility issues when combining LINQ with other technologies.
- Risk of Overusing Features: Overusing advanced LINQ features, such as complex joins, nested queries, and groupings, can result in less readable and maintainable code. It's like adding too many ingredients to a recipe, especially in scenarios where simpler code is more appropriate.
- Tooling Limitations: While Visual Studio provides good support for LINQ, not all development tools may offer the same level of assistance. It's like having a high-tech tool in a low-tech environment; there might be some limitations.
Despite these potential disadvantages, LINQ remains a powerful and widely used tool in the .NET ecosystem. The decision to use LINQ should be based on the specific needs of the project and the development team's familiarity with the technology.Learn Linq Syntax in the next tutorial.